Tuesdays 9:30AM – noon, in 370 Jay St Room 450
Contact
My email: tom.igoe@nyu.edu
You can book office hour appointments on my office hours calendar. You’ll need to sign in with your NYU login to do so. Generally my office hours are on Tuesday, Wednesday, and Thurday, during times when most first years have few or no classes. I may have to shift from week to week due to other school commitments, however. Watch the link above for the latest. Please book no more than one a week, so that I can serve you all as equally as possible.
Outside of office hours, I generally work from 9 AM to 5 PM NYC time Monday-Friday. I’m usually on campus Mon – Thurs, and work offsite Fridays. If you contact me outside of working hours, I’ll try to get back to you as soon as my next working time allows.
Class Blogs
Please fill in your blog link and other details here. (requires NYU login). I learn a lot about your progress in this class from your blogs, and it improves the quality of the class. So post frequently. Please set up a category/menu for this class and submit the link for that category, not to your whole blog. I don’t have a Notion account, so if you use Notion, please publish the pages publicly so I can see them. NYU WordPress is another viable option.
Useful links
- The week-by-week syllabus. When in doubt, check here.
- Arduino Language Reference – When you can’t remember or haven’t seen an Arduino function before, look it up here.
- The Nano 33 IOT page – useful for the pin functions in the Technical Specification
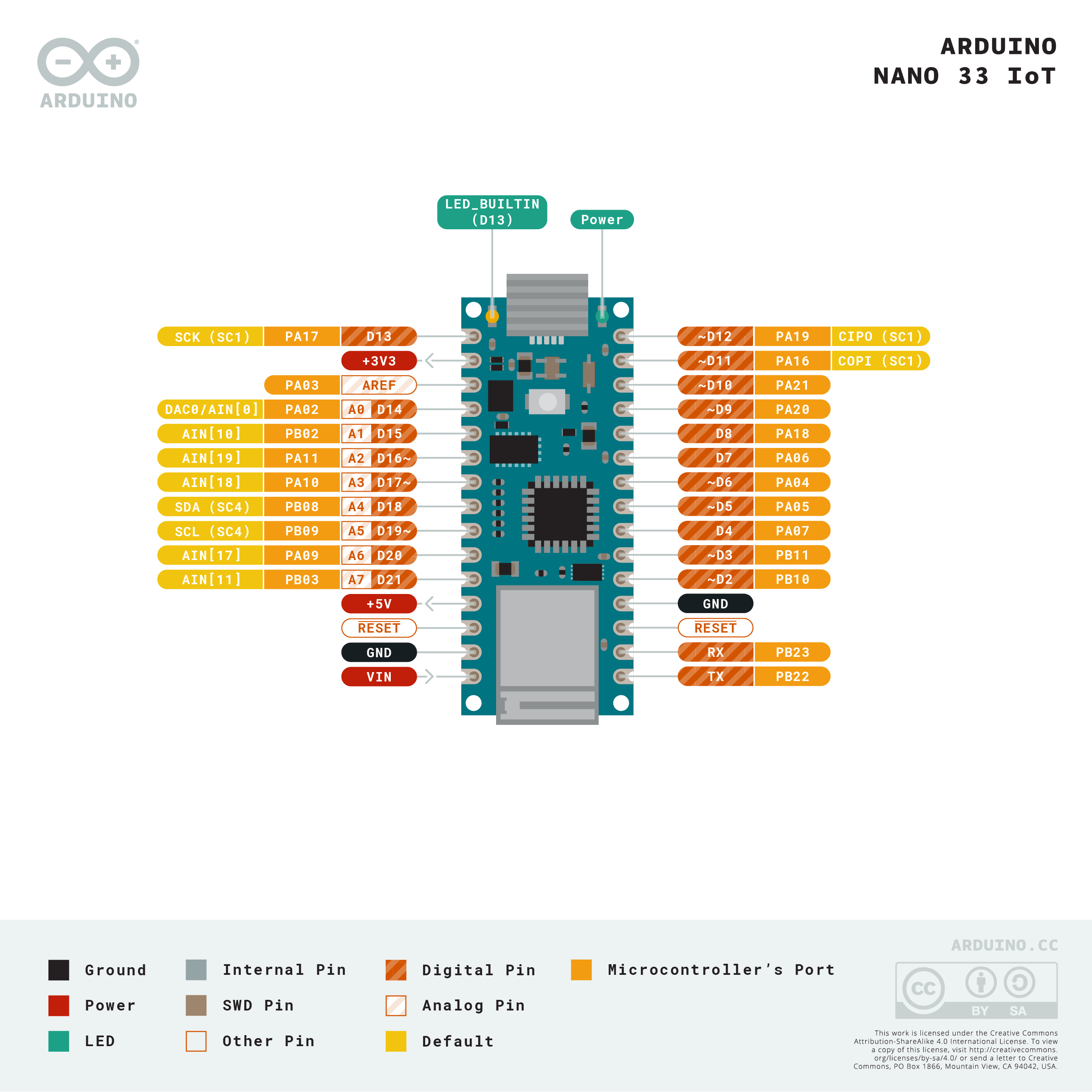
- Tom’s Site
- Tom’s gitHub repository
- Rob Ives, formerly Flying Pig, a great site for simple mechanisms
MIDI repository referenced on 24 Sept 2024 class
Code from 24 Sept 2024
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 | /* MIDI Note player with a button sends a MIDI note on message when the button is pressed. Sends a MIDI noteoff message when the button is released. */ #include <MIDIUSB.h> // digital pin 2 has a pushbutton attached to it. Give it a name: int pushButton = 2; // variable for the state of the button last time through the loop: int lastState = 0; // current note being played: byte currentNote = 69; // the setup routine runs once when you press reset: void setup() { // initialize serial communication at 9600 bits per second: Serial.begin(9600); // make the pushbutton's pin an input: pinMode(pushButton, INPUT); } // the loop routine runs over and over again forever: void loop() { // read the input pin: int buttonState = digitalRead(pushButton); // if the button is changed: if (buttonState != lastState) { // take action if (buttonState == LOW) { // button release // send a MIDI noteoff message midiCommand(0x80, currentNote, 0); Serial.println("release"); } else { // button press // generate a new note pitch: currentNote = random(30) + 60; // send a MIDI noteon message: midiCommand(0x90, currentNote, 127); Serial.println("press"); } } // save the current button state for next time // through the loop: lastState = buttonState; } // send a 3-byte midi message void midiCommand(byte cmd, byte data1, byte data2) { // First parameter is the event type (top 4 bits of the command byte). // Second parameter is command byte combined with the channel. // Third parameter is the first data byte // Fourth parameter second data byte midiEventPacket_t midiMsg = { cmd >> 4, cmd, data1, data2 }; MidiUSB.sendMIDI(midiMsg); } |